How To Change Items In A List In Python
- Python List is a mutable sequence.
- We tin can create a List past placing elements within a foursquare subclass.
- The listing elements are separated using a comma.
- We can create nested lists.
- The list is an ordered collection. So it maintains the guild in which elements are added.
- We can admission list elements using index. It also supports negative index to refer elements from end to start.
- We tin unpack list elements to comma-separated variables.
- Python List can have duplicate elements. They also allow None.
- Listing support 2 operators: + for concatenation and * for repeating the elements.
- Nosotros can piece a list to create another listing from its elements.
- Nosotros can iterate over listing elements using the for loop.
- We can use "in" operator to cheque if an item is present in the list or non. Nosotros tin also apply "not in" operator with a list.
- A listing is used to shop homogeneous elements where we desire to add/update/delete elements.
Creating a Python List
Python list is created by placing elements within square brackets, separated with a comma.
fruits_list = ["Apple", "Banana", "Orange"]
Nosotros tin can continue different types of elements in a list.
random_list = [1, "A", object(), x.55, True, (1, 2)]
We tin can also have nested lists.
nested_list = [ane, [2, 3], [4, 5, half dozen], 7]
We can create an empty list by having no elements inside the square brackets.
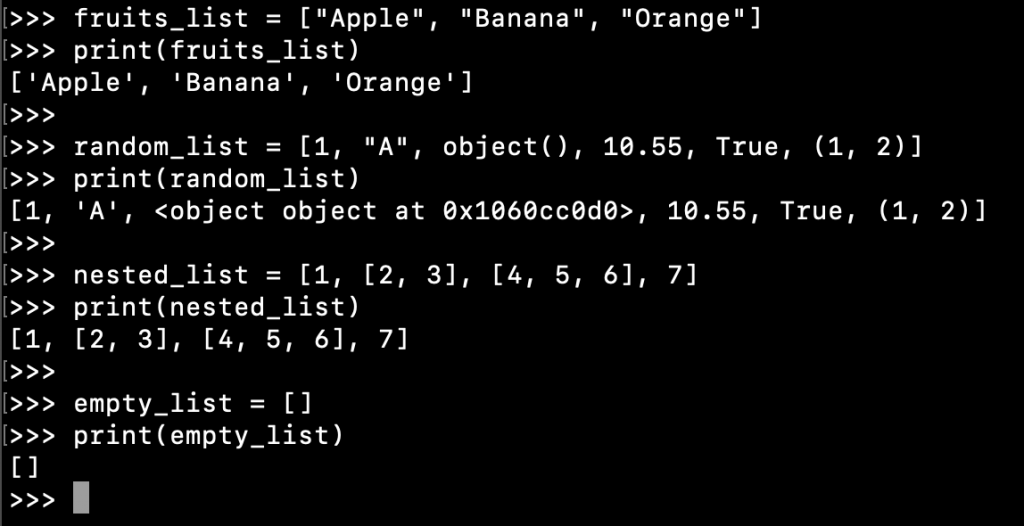
Accessing List Elements
We tin access list elements using index. The index value starts from 0.
>>> vowels_list = ["a", "east", "i", "o", "u"] >>> vowels_list[0] 'a' >>> vowels_list[four] 'u'
If the index is not in the range, IndexError is raised.
>>> vowels_list[forty] Traceback (most recent call final): File "<stdin>", line one, in <module> IndexError: list index out of range >>>

Nosotros can as well laissez passer a negative index value. In this case, the element is returned from the terminate to start. The valid alphabetize value range is from -1 to -(list length).
This is useful when nosotros want a specific element quickly, such as the last element, second terminal chemical element, etc.
>>> vowels_list = ["a", "e", "i", "o", "u"] >>> vowels_list[-1] # last element 'u' >>> vowels_list[-2] # 2d final element 'eastward' >>> vowels_list[-5] 'a'
Accessing Nested List Elements
We can access nested list elements using the nested indexes. Let's understand this with some simple examples.
nested_list = [1, [ii, 3], [iv, 5, 6], 7] # first element in the nested sequence at index 1 print(nested_list[1][0]) # second element in the nested sequence at index 1 print(nested_list[one][1]) # third element in the nested sequence at alphabetize 2 print(nested_list[2][2])
The nested element can be any other sequence also that supports index-based access. For instance, the result will be the same for the nested list [1, (ii, 3), (4, 5, 6), 7].
We can use negative indexes with nested lists too. The above code snippet can be rewritten as follows.
nested_list = [1, (2, 3), (iv, 5, 6), 7] # first element in the nested sequence at third terminal index print(nested_list[-3][0]) # last element in the nested sequence at 3rd last index print(nested_list[-3][-1]) # last element in the nested sequence at second last index print(nested_list[-2][-1])

Updating a Listing
We tin can apply the assignment operator to change the list value at the specified index.
>>> my_list = [1, 2, iii] >>> my_list[1] = x >>> my_list [1, ten, 3] >>>
Iterating through a Listing
Nosotros tin can use the for loop to iterate over the elements of a listing.
>>> my_list = [ane, 2, three] >>> for ten in my_list: ... print(10) ... ane 2 three >>>
If y'all want to iterate over the listing elements in the reverse order, y'all can employ reversed() built-in function.
>>> my_list = [1, two, three] >>> for 10 in reversed(my_list): ... print(x) ... 3 2 1 >>>
Cheque if an item exists in the list
We can use "in" operator to bank check if an item is present in the list or non. Similarly, we can also use "non in" operator with the list.
>>> my_list = [one, two, three] >>> >>> i in my_list True >>> 10 in my_list Simulated >>> ten not in my_list True >>> 1 not in my_list False >>>
Deleting a Listing
We tin employ "del" keyword to delete a list alphabetize or the complete list itself.
>>> my_list = [1, 2, three] >>> del my_list[1] >>> my_list [i, three] >>> >>> del my_list >>> my_list Traceback (most recent call last): File "<stdin>", line 1, in <module> NameError: proper noun 'my_list' is not defined >>>
Slicing a List
We can use slicing to create a new list from the elements of a listing. This is useful in creating a new list from a source list.
The slicing technique contains two indexes separated using a colon. The left alphabetize is included and the correct alphabetize is excluded from the result.
list_numbers = [1, 2, three, four, five, 6, seven, 8] impress(list_numbers[one:3]) print(list_numbers[:iv]) print(list_numbers[5:]) print(list_numbers[:-five])

Listing Concatenation (+ operator)
We tin concatenate multiple lists of elements to create a new list using the + operator.
>>> l1 = [ane] >>> l2 = [2, 3] >>> l3 = [4, "five", (6, 7)] >>> >>> l1 + l2 + l3 [1, 2, three, 4, '5', (six, 7)] >>>
Repeating Listing Elements (* operator)
Python List also supports * operator to create a new list with the elements repeated the specified number of times.
>>> l1 = [i, 2] >>> >>> l1 * 3 [i, two, 1, two, 1, 2] >>>
Python List Length
Nosotros can get the length or size of the list using the built-in len() function.
>>> list_numbers = [i, two, 3, 4] >>> len(list_numbers) 4
The built-in list() Constructor
We can create a list from iterable using the born list() constructor. This function accepts an iterable argument, so nosotros can pass String, Tuple, etc.
>>> l1 = listing("ABC") >>> l1 ['A', 'B', 'C'] >>> >>> l1 = list((ane, 2, iii)) >>> >>> l1 [ane, 2, 3] >>>
Python Listing Functions
Let's expect at some of the functions present in the list object.
ane. append(object)
This function is used to suspend an element to the end of the list.
>>> list_numbers = [ane, 2, 3, iv] >>> list_numbers.append(five) >>> impress(list_numbers) [1, 2, three, 4, 5]
ii. index(object, start, end)
This role returns the first index of the object in the list. If the object is non found, and then ValueError
is raised.
The start and end are optional arguments to specify the alphabetize from where to start and cease the search of the object.
>>> list_numbers = [one, 2, 1, ii, 1, 2] >>> >>> list_numbers.index(i) 0 >>> list_numbers.index(1, i) 2 >>> list_numbers.index(one, 3, ten) 4 >>> list_numbers.index(x) Traceback (almost recent telephone call last): File "<stdin>", line i, in <module> ValueError: x is not in list >>>
3. count(object)
This function returns the number of occurrences of the object in the list.
>>> list_numbers = [1, two, one, 2, 1, two] >>> list_numbers.count(ii) 3 >>> list_numbers.count(1) 3
four. reverse()
This function reverses the listing elements.
>>> list_numbers = [1, two, three] >>> list_numbers.reverse() >>> print(list_numbers) [iii, 2, one]
5. articulate()
This office removes all the elements from the list.
>>> list_numbers = [one, 2, 5] >>> list_numbers.clear() >>> print(list_numbers) []
6. copy()
This function returns a shallow copy of the listing.
>>> list_items = [1, 2, iii] >>> tmp_list = list_items.re-create() >>> print(tmp_list) [one, 2, 3]
vii. extend(iterable)
This function appends all the elements from the iterable to the finish of this listing. Some of the known iterable in Python are Tuple, List, and String.
>>> list_num = [] >>> list_num.extend([1, ii]) # list iterable statement >>> print(list_num) [1, two] >>> list_num.extend((3, 4)) # tuple iterable statement >>> print(list_num) [1, 2, three, 4] >>> list_num.extend("ABC") # string iterable argument >>> print(list_num) [i, ii, 3, 4, 'A', 'B', 'C'] >>>
8. insert(index, object)
This method inserts the object only earlier the given index.
If the index value is greater than the length of the list, the object is added at the end of the list.
If the index value is negative and not in the range, and so the object is added at the beginning of the list.
>>> my_list = [1, ii, 3] >>> >>> my_list.insert(1, 'X') # insert only before index ane >>> print(my_list) [one, '10', 2, three] >>> >>> my_list.insert(100, 'Y') # insert at the end of the list >>> impress(my_list) [1, 'X', 2, 3, 'Y'] >>> >>> my_list.insert(-100, 'Z') # negative and not in range, so insert at the start >>> print(my_list) ['Z', 1, 'X', ii, 3, 'Y'] >>> my_list.insert(-ii, 'A') # negative and in the range, and so insert before second final chemical element >>> impress(my_list) ['Z', i, 'Ten', two, 'A', 3, 'Y'] >>>
ix. pop(index)
This part removes the element at the given index and returns it. If the index is not provided, the last element is removed and returned.
This function raises IndexError if the list is empty or the index is out of range.
>>> my_list = [i, two, 3, 4] >>> >>> my_list.popular() 4 >>> my_list [1, ii, 3] >>> my_list.pop(1) two >>> my_list [i, three] >>> my_list.pop(-1) iii >>> my_list [1] >>> my_list.pop(100) Traceback (near recent call terminal): File "<stdin>", line one, in <module> IndexError: popular alphabetize out of range >>>
10. remove(object)
This function removes the outset occurrence of the given object from the listing. If the object is not found in the list, ValueError is raised.
>>> my_list = [1,ii,3,1,two,three] >>> my_list.remove(ii) >>> my_list [i, 3, 1, 2, 3] >>> my_list.remove(xx) Traceback (most recent phone call terminal): File "<stdin>", line one, in <module> ValueError: list.remove(x): x not in list >>>
eleven. sort(key, reverse)
This function is used to sort the list elements. The list elements must implement __lt__(self, other) part.
We can specify a function proper name every bit the key to be used for sorting. This way nosotros can ascertain our ain custom office to use for sorting list elements.
The reverse accepts a boolean value. If it's True, then the listing is sorted in the reverse order. The default value of reversed is False and the elements are sorted in the natural order.
>>> list_num = [ane, 0, 3, iv, -i, 5, 2] >>> list_num.sort() >>> list_num [-1, 0, 1, 2, iii, 4, v] >>> list_num.sort(opposite=True) >>> list_num [5, iv, iii, ii, 1, 0, -1] >>>
Listing vs Tuple
- The listing is a mutable sequence whereas Tuple is immutable.
- The list is preferred to store the same types of data types where we need to add/update them.
- A list requires more retentivity than Tuple because it supports dynamic length.
- Iterating over a list is slightly more time taking than a Tuple because its elements are non required to exist stored in contiguous memory locations.
Conclusion
Python List is a mutable sequence. It provides various functions to add together, insert, update, remove its elements. We can create a listing from other iterable elements using the built-in list() constructor.
References:
- Python Listing Data Structure
- list() congenital-in function
Source: https://www.askpython.com/python/list/python-list
Posted by: dennisalannow.blogspot.com
0 Response to "How To Change Items In A List In Python"
Post a Comment